Dive Board
-
Guide to Build a Weather App with ReactJS and OpenWeatherMap API
Guide to Build a Weather App with ReactJS and OpenWeatherMap API
by bookerrjanee on Feb 22nd, 2023 11:59 AM
In this tutorial, we'll be building a weather app using ReactJS and the OpenWeatherMap API. A weather app can be a useful and practical project to work on, as it provides real-world functionality and can be customized in many ways. ReactJS is a popular JavaScript library for building user interfaces, and it's well-suited for creating dynamic and responsive web applications like a weather app.
Throughout this tutorial, we'll cover the steps involved in building the weather app from scratch. We'll start by setting up the project with create-react-app, then move on to creating the Weather component that will fetch and display the weather data. We'll also cover how to parse the data received from the API and format it for display. Additionally, we'll add user input functionality that allows the user to search for weather information for any location they choose. Lastly, we'll implement error handling to handle any issues that may arise when fetching weather data from the API.
By the end of this tutorial, you'll have a functional weather app built with ReactJS that can fetch and display weather data for any location in the world. Let's get started!
Setting up the project
In this section, we'll cover the steps involved in setting up the project for our weather app. We'll start by creating a new React app using create-react-app, then we'll install the necessary dependencies for making API calls to the OpenWeatherMap API. Finally, we'll set up the project structure to organize our code in a clear and logical way.
Creating a new React app using create-react-app:
To create a new React app using create-react-app, open up your terminal and navigate to the directory where you want to create your app. Once you're there, run the following command:
npx create-react-app weather-app
This will create a new React app called "weather-app" in the current directory. Once the installation is complete, you can navigate to the new app directory by running:
cd weather-app
With this command, you're now inside the new React app's directory.
Installing necessary dependencies:
Next, we'll need to install a few dependencies to make API calls to the OpenWeatherMap API. We'll use axios, which is a popular HTTP client for making API calls in JavaScript. To install axios, run the following command in your terminal:
npm install axios
This will install axios and its dependencies in your project.
Setting up the project structure:
To keep our code organized, we'll create a new directory called "components" in the src/ directory. This is where we'll store our React components. To create the directory, run the following command:
mkdir src/components
Next, create a new file inside the src/components/ directory called "Weather.js". This will be the main component for our weather app. To create the file, run the following command:
touch src/components/Weather.js
With these steps, we've set up the project for our weather app. In the next section, we'll start building the Weather component.
Creating the Weather component
In this section, we'll start building the Weather component, which will fetch and display weather data from the OpenWeatherMap API. We'll cover the purpose and structure of the Weather component, implement its layout using CSS, and add state to the component to fetch data from the API.
Explanation of the Weather component's purpose and structure:
The Weather component is the main component of our weather app. It will display the current weather conditions, as well as the daily forecast for the next few days for a given location. The Weather component will use the OpenWeatherMap API to fetch weather data, and will then display that data on the page.
The structure of the Weather component will consist of a form for the user to enter a location, and a section for displaying the weather data. The form will allow the user to input a city name, and when the form is submitted, the Weather component will fetch the weather data for that location from the API.
Implementing the component's layout using CSS:
To implement the layout of the Weather component, we'll use CSS. We'll start by creating a new file inside the src/ directory called "Weather.css". This is where we'll write our CSS code for styling the Weather component.
We'll begin by creating a basic layout for the Weather component. We'll use a container element to hold the form and the weather data display section. Inside the container, we'll have a form element with an input field for the user to enter a city name, and a submit button to submit the form. We'll also have a section element for displaying the weather data. The CSS code for this layout might look something like this:
.weather-container {
display: flex;
flex-direction: column;
align-items: center;
}
.weather-form {
display: flex;
flex-direction: row;
align-items: center;
margin-bottom: 1rem;
}
.weather-form input[type="text"] {
margin-right: 1rem;
padding: 0.5rem;
font-size: 1rem;
}
.weather-form button {
padding: 0.5rem 1rem;
font-size: 1rem;
}
.weather-data {
display: flex;
flex-direction: column;
align-items: center;
}
This CSS code sets up a basic layout for the Weather component, with a container element that holds a form for the user to input a city name, and a section for displaying the weather data.
Adding state to the component and fetching data from the OpenWeatherMap API:
Next, we'll add state to the Weather component, which will allow us to fetch data from the OpenWeatherMap API and display it on the page. We'll use the useState hook in React to manage the component's state.
To fetch data from the OpenWeatherMap API, we'll use axios to make an API call to the API endpoint for the city the user inputs. We'll use the API key provided by OpenWeatherMap to authenticate our request.
Here's what the Weather component's code might look like at this stage:
import React, { useState } from "react";
import axios from "axios";
import "./Weather.css";
const Weather = () => {
const [weatherData, setWeatherData] = useState(null);
const [cityName, setCityName] = useState("");
const handleSubmit = async (e) => {
e.preventDefault();
const API_KEY = "your_api_key_here";
const url = `https://api.openweathermap.org/data/2.5/weather?q=${cityName}&appid=${API_KEY}`;
const response = await axios.get(url);
setWeatherData(response.data);
};
return (
{weatherData && (
{weatherData.name}
{weatherData.weather[0].description}
Temperature: {(weatherData.main.temp - 273.15).toFixed(1)}°C
Humidity: {weatherData.main.humidity}%
)}
);
};
export default Weather;
In this code, we define the Weather component, and use the useState hook to define two pieces of state: weatherData and cityName. weatherData will store the weather data fetched from the OpenWeatherMap API, and cityName will store the name of the city entered by the user in the form.
The handleSubmit function is called when the user submits the form. This function prevents the default form submission behavior, constructs the API request URL with the city name entered by the user and the API key, and makes a GET request using axios to the OpenWeatherMap API. If the request is successful, the weather data is stored in the weatherData state variable using the setWeatherData function.
In the JSX code, we use the weatherData state variable to conditionally render the weather data section of the component. If weatherData is not null, we display the weather data using the properties returned by the OpenWeatherMap API.
At this stage, the Weather component can fetch weather data from the OpenWeatherMap API and display it on the page. However, there's still some work to do to make the component more useful and user-friendly. We'll cover this in the next section.
Displaying the weather data
When we receive the weather data from the API, it's in a JSON format. To display this data, we need to extract the relevant information and format it as required. In our code, we have already extracted the city name, weather description, temperature, and humidity from the data. However, the temperature is in Kelvin, which is not the most user-friendly format. To display it in Celsius, we need to convert it.
We can create a helper function that converts temperatures from Kelvin to Celsius. Here's what the code might look like:
const kelvinToCelsius = (temp) => {
return (temp - 273.15).toFixed(1);
};
We can use this function to format the temperature data in the JSX code:Temperature: {kelvinToCelsius(weatherData.main.temp)}°C
Similarly, we can create helper functions for other data formatting tasks. For example, we can format the date and time using the built-in Date object, or we can format the wind speed to show it in miles per hour.
Once we have formatted the data, we can display it in the Weather component. We can use conditional rendering to show the data only when it's available. Here's what the code might look like:
{weatherData && (
{weatherData.name}
{weatherData.weather[0].description}
Temperature: {kelvinToCelsius(weatherData.main.temp)}°C
Humidity: {weatherData.main.humidity}%
)}
In this code, we are using the && operator to conditionally render the weather data section only when weatherData is not null. When the data is available, we display the city name, weather description, temperature, and humidity.
By formatting and displaying the weather data in a user-friendly format, we have made the Weather component more useful and accessible. However, there's still room for improvement. In the next section, we'll cover how to add more features to the component to make it even more useful.
Adding user input
In the previous sections, we built a Weather component that displays weather data for a fixed location. In this section, we'll add a feature that allows the user to enter a location of their choice.
To do this, we need to create a form that allows the user to enter a city name. We can add this form to the Weather component by modifying the JSX code as follows:
In this code, we've added a form element that calls the handleSubmit function when the user submits the form. The input element allows the user to enter a city name, and we are storing the value of this input in the cityName state variable using the onChange event. We've also added a "Search" button to submit the form.
Now that we're storing the user's input in the component's state, we need to update the API call to use this input. We can do this by modifying the URL that we pass to the axios.get function in the handleSubmit function:
const url = `https://api.openweathermap.org/data/2.5/weather?q=${cityName}&appid=${API_KEY}`;
Now, when the user submits the form, the handleSubmit function will use the user's input to fetch weather data for the specified location.
However, there's one more thing we need to do. When the user submits the form, we want to clear the input field so that it's ready for the next search. We can do this by adding the following line to the handleSubmit function:
setCityName("");
This line sets the cityName state variable to an empty string, which clears the input field.
With these changes, our Weather component now allows the user to search for weather data for any location they choose. By adding this feature, we've made our component much more useful and versatile.
Handling errors
In this section, we'll add error handling to our Weather component to handle situations where the API call fails. We'll also display an error message to the user to let them know that something went wrong.
To start, we need to modify the handleSubmit function to handle errors that might occur during the API call. We can do this by adding a try...catch block around the axios.get call, like so:
try {
const response = await axios.get(url);
setWeatherData(response.data);
} catch (error) {
console.log(error);
}
In this code, we're wrapping the API call in a try block. If an error occurs, the code in the catch block will be executed. For now, we're just logging the error to the console, but in the next step, we'll use this error to display a message to the user.
Next, we'll modify the JSX code to display an error message when an error occurs. We can do this by adding a new state variable called error and modifying the handleSubmit function to set this variable when an error occurs:
const [error, setError] = useState(null);
const handleSubmit = async (e) => {
e.preventDefault();
const API_KEY = "your_api_key_here";
const url = `https://api.openweathermap.org/data/2.5/weather?q=${cityName}&appid=${API_KEY}`;
try {
const response = await axios.get(url);
setWeatherData(response.data);
setError(null);
} catch (error) {
console.log(error);
setError("Error fetching weather data. Please try again.");
}
};
In this code, we've added a new state variable called error that starts off as null. In the handleSubmit function, we set this variable to an error message when an error occurs. We're also setting the weatherData state variable to null when an error occurs to clear any previously displayed weather data.
Finally, we can modify the JSX code to display the error message when the error state variable is not null. We can do this by adding a new div element below the form:
{error &&{error}}
In this code, we're using a conditional rendering to display the div element only if the error state variable is not null. If there is an error, the div element will display the error message.
With these changes, our Weather component now has error handling that displays an error message to the user when something goes wrong with the API call. By doing this, we've made our component more user-friendly and resilient.
Conclusion
In this article, we've built a simple weather app using ReactJS and the OpenWeatherMap API. We started by setting up the project, creating the Weather component, and displaying the weather data. We then added user input and error handling to make our app more robust and user-friendly.
We've covered many important concepts in this article, including working with APIs, using state and props in React, and handling user input and errors. With the knowledge gained from building this app, you'll be able to tackle more complex React projects and APIs.
If you're looking to build a React app or need help with your existing React project, hire reactjs developers. They can help you build high-quality apps that meet your specific requirements and exceed your expectations.
We hope you found this article helpful and informative. Thanks for reading!bookerrjanee
Posts: 3
Joined: 09.02.2023
Re: Guide to Build a Weather App with ReactJS and OpenWeatherMap API
by seolinks786 on Feb 22nd, 2023 20:49 PM
[color=#1a202c][font=Poppins, sans-serif][size=3]It is a stunning option for beginners, and snorkel review 2023 Spectra Low Volume 2 Window Dive Mask has everything you require.[/size][/font][/color]
seolinks786
Posts: 1253
Joined: 28.11.2022
Re: Guide to Build a Weather App with ReactJS and OpenWeatherMap API
by soaptoday on Mar 24th, 2023 11:51 AM
[color=#5f6368][size=2][font=arial, sans-serif]YTMP4[/font][/size][/color][color=#4d5156][size=2][font=arial, sans-serif] is the [/font][/size][/color][color=#5f6368][size=2][font=arial, sans-serif]best[/font][/size][/color][color=#4d5156][size=2][font=arial, sans-serif] online video downloader. Here you can download videos from more than 100 websites: Facebook, Instagram, Twitter, Vimeo etc.[/font][/size][/color]
soaptoday
Posts: 40
Joined: 10.10.2022
Re: Guide to Build a Weather App with ReactJS and OpenWeatherMap API
by AmandaReece on Apr 10th, 2023 08:37 AM
The "Guide to Build a Weather App with ReactJS and OpenWeatherMap API" is a step-by-step tutorial that teaches developers how to create a weather app using ReactJS and the OpenWeatherMap API. Here you follow this Node js Development Services and learn more new tips for developers. The guide covers everything from setting up the project to displaying weather data in the app.
AmandaReece
Posts: 119
Joined: 20.01.2023
Re: Guide to Build a Weather App with ReactJS and OpenWeatherMap API
by Carbide Inserts on Apr 23rd, 2023 05:07 AM
Cemented Carbide Inserts
The products is as beautiful and striking as it is mechanically distinguished. You will TPMT Carbide Inserts find what is good value for money.Not Tungsten Steel Inserts to mention, new Identify cheap product defined milling inserts by the last appearance of sophistication SNMG Cermet Inserts appear as icons in Carbide Drilling Inserts your collection.Carbide Inserts
Posts: 49
Joined: 21.04.2023
best snorkel mask anti fog
by sratim tv on Aug 5th, 2023 14:20 PM
With safety-reinforced anti-fog lenses, it is one of the best snorkel mask anti fog. Ploy-carbon coating on lenses enables the fog-resistance phenomena. Additionally, it incorporates anti-ultraviolet characteristics to shield the eyes from the sun's rays. The 1800 field of vision has been included into its design on both sides. You will be able to enjoy the underwater world more because to the wide field of vision.
sratim tv
Posts: 35
Joined: 17.07.2023
RECENT TOPICS
شركة عزل فوم بالرياض
شركة عزل اسطح بالرياض
Hybrid Crypto Exchanges Development: Best Practices Maticz Follows
STATISTICS
Total posts: 216015
Total topics: 56867
Total members: 48285
Newest member: Daniel G.
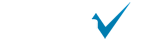
Rencontrez la famille Durovis Dive et transformer votre appareil mobile en un casque de réalité virtuelle. jeux d'expérience et 360 vidéos ° en VR sur votre propre smartphone ou tablette.
Modes de paiement acceptés

